1. 숫자 맞추기 게임 만들기
게임 규칙: 컴퓨터가 숫자를 생각함 -> 사용자가 난이도를 고름 -> 사용자가 숫자를 입력함 -> 높으면 높다고, 낮으면 낮다고 컴퓨터가 알려줌 -> 쉬운레벨은 10번만에, 어려운 레벨은 5번만에 맞춰야 함
1.1. 사용된 개념 - 유효범위, 전역변수
유효 범위(scope)
- 지역 범위(local scope): 함수 내에 존재. 함수 내부에 있을 때만 사용할 수 있음.
- 전역 범위(global scope): 파일 내부에서 정의되었기 때문. 함수 외부에서 존재. 함수 내부에서 얼마나 중첩되었는지는 상관없음.
- 네임스페이스: 이름을 지정한 모든 공간에 이름공간이 있는데, 그 네임스페이스 특정 범위 안에서 유효하다
- for, if, while loop는 별도의 지역범위를 만드는 것으로 간주되지 않는다.
- 전역변수를 수정하는 방법전역변수를 되도록이면 수정하지 말 것. 대신 return 사용하기
- 파이썬의 명명규칙은 변수를 대문자로 바꾸는 것이다.
예) PI = 3.14 / URL = www.google.com
코드 예시
<python />
enemies = 1
def increase_enemies():
print("enemies inside function")
return enemies + 1
enemies = increase_enemies()
1.2. 내가 짠 코드
<python />
from art import logo
print(logo)
import random
hard = 5
easy = 10
def play_game(level):
random_number = random.randint(0, 100)
while level > 0:
guess_number = int(input("guess number!: "))
if guess_number == random_number:
print(f"you win this game! the answer is {random_number}")
replay_game = input("Do you wanna play game again? (y/n): ")
if replay_game == 'y':
return hello()
else:
print("see you next time")
break
elif guess_number > random_number:
print("Too high")
level -= 1
elif guess_number < random_number:
print("Too low")
level -= 1
if level == 0:
print(f"you lose this game! the answer is {random_number}")
replay_game = input("Do you wanna play game again? (y/n")
if replay_game == 'y':
return hello()
else:
print("goodbye!")
def game_start(user_choice):
if user_choice == "hard":
print(f"you have {hard} chance to play game.")
play_game(hard)
elif user_choice == "easy":
print(f"you have {easy} chance to play game.")
play_game(easy)
else:
print("please write on 'hard' or 'easy'")
def hello():
print("welcome to the guessing game!")
print("you can choose the level of game")
print("hard level or easy level")
user_choice = input("what do you want to choose? hard or easy: ")
game_start(user_choice)
hello()
로고
<python />
logo = """
___ _
/ _ \_ _ ___ ___ ___ _ __ _ _ _ __ ___ | |__ ___ _ __
/ /_\/ | | |/ _ \/ __/ __| | '_ \| | | | '_ ` _ \| '_ \ / _ \ '__|
/ /_\\| |_| | __/\__ \__ \ | | | | |_| | | | | | | |_) | __/ |
\____/ \__,_|\___||___/___/ |_| |_|\__,_|_| |_| |_|_.__/ \___|_|
"""
1.3. 코드 실행 사진
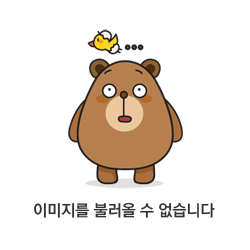
디자인을 대충 포기하고 간단히 만들었다.
수업시간에 만들면 약간 눈치보여서 쉬는시간에 간단히 만들어 봤다.
함수로 만드려고 노력했는데 잘 나와서 기분 좋음!
1.4. 참고 코드- 유데미 안젤라 파이썬 강의
<python />
from random import randint # import random을 사용할 수 도 있으나 이렇게도 쓸 수 있다.
from art import logo
hard_level_turns = 5
easy_level_turns = 10
def check_answer(guess, answer, turns):
if guess > answer:
print("Too high")
return turns - 1
elif guess < answer:
print("Too low")
return turns - 1
else:
print(f"You got it! The answer was {answer}")
def set_difficulty():
level = input("Choose a difficulty. easy or hard: ")
if level == "easy":
return easy_level_turns
else:
return hard_level_turns
def game():
print(logo)
# 1부터 100까지 랜덤 넘버 고르기
print("Welcome tho the guessing game!")
print("I'm thinking of a number between 1 and 100")
answer = randint(1, 100)
turns = set_difficulty()
guess = 0
while guess != answer:
print(f"You have {turns} attempts remaning to guess the number.")
guess = int(input("Make a guess: "))
turns = check_answer(guess, answer, turns)
if turns == 0:
print("You've run out of guesses, you lose.")
return
elif guess != answer:
print("Guess again.")
game()
와방깔끔.. 함수사용방법을 더 공부해야겠다.
'개발공부 > Python' 카테고리의 다른 글
[파이썬] while문으로 구구단 찍기, 별찍기 (0) | 2023.04.21 |
---|---|
파이썬으로 커피 자판기 만들기 (1) | 2023.04.16 |
[파이썬] 집합(=세트, Sets)_ 기능, 메서드 (1) | 2023.04.13 |
[파이썬] 튜플(Tuple) (0) | 2023.04.13 |
[파이썬] 리스트(List)_메서드, 스택, 큐, 컴프리헨션 (0) | 2023.04.13 |