4/26일 공부&작성한 코드
4/27일 암호화, 복호화 내용 추가
목차
개발상황
수업시간에 해본 함수 연습 코드
---------------------------
로그인창 개발완료보고서
로그인창 순서도
로그인창 코드
로그인창 코드구현사진
만난 오류 및 해결방안
오늘 새로 배운 내용
더 해야할 점
개발상황
오늘은 숫자야구를 함수로 만들기와 회원가입, 로그인 화면을 구축하는 코드를 함수로 만드는 시간을 가졌다.
완료한 후 디자인이 맘에들지 않아 열심히 수정했는데, 결과물은 만족하지만 나중에 GUI를 배우면 그때 열중해보는 시간을 가져보려고 한다. 아직 파이썬 콘솔에서 꾸며보려고하니 한계가 느껴진다.
최대한 함수를 써보려고 했는데 맞게 한 건지 궁금하다. 다른 사람들의 코드를 빨리 보고 비교해보고 싶다.
수업시간에 해본 함수연습
1. 숫자가 아니면 물어보는 함수 만들기(재귀함수 사용)
def str_to_int(user_input):
if user_input.isdigit() == True:
return user_input
else:
retry = input("다시 입력하세요: ")
return str_to_int(retry)
question = input("숫자를 입력하세요: ") #사용자가 입력함
a = str_to_int(question)
print(a)
2. 계산기 만들어보기
#함수명 add이고, int로 2개의 인자를 받아서 둘을 더해준 값을 반환하는 함수 만들기
def add(a, b):
return a+b
def div(a, b):
return a/b
def sub(a, b):
return a-b
def multiple(a, b):
return a*b
def square(a, b):
return a**b
def remainder(a,b):
return a%b
def quotient(a,b):
return a//b
a, b = input("숫자를 띄어쓰기로 입력하세요: ").split()
a = int(a)
b = int(b)
print(a, "+", b, "=", add(a, b))
print(a, "/", b, "=", div(a, b))
print(a, "-", b, "=", sub(a, b))
print(a, "*", b, "=", multiple(a, b))
print(a, "**", b, "=", square(a, b))
print(a, "%", b, "=", remainder(a, b))
print(a, "//", b, "=", quotient(a, b))
2.1. 계산기 0 넣지말게 만들어보기
def not_include_zero(a, b):
if a == 0 or b == 0:
print("0을 입력하지 마세요. 다시 입력하세요.")
return True
else:
return False
i = True
while i != False:
a = int(input("첫번째 숫자 입력: "))
b = int(input("두번째 숫자 입력: "))
i = not_include_zero(a, b)
print(a, "+", b, "=", add(a, b))
print(a, "/", b, "=", division(a, b))
print(a, "-", b, "=", sub(a, b))
print(a, "*", b, "=", multiple(a, b))
print(a, "**", b, "=", square(a, b))
print(a, "%", b, "=", remainder(a, b))
print(a, "//", b, "=", quotient(a, b))
3. default 값 넣기(키워드 매개변수)
문제:
#함수명 str_concat이고, 인자로 2개의 str을 입력받아 둘을 더해주고 값을 반환해주는 함수를 작성하세요. 단 두번째 인자가 입력받지 않으면 default로 "Hello!"로 작동하도록 하세요.
단, 사용자는 str을 제외한 값은 넣지 않습니다.
def str_concat(a, b = "Hello"):
return a + b
print(str_concat("안녕", "나야나"))
#안녕나야나
4. 리스트 뒤집기
함수명 reverse이고, 인자(list_)로 특정 길이의 리스트를 1개 받아서 리스트를 출력해주는 함수를 작성하세요. 단, 인자가 없을 경우는 없습니다. isinstance쓰지마세요! 무조건 리스트 받을거에요.
def reverse(list_2):
empty_list = []
for i in list_2[::-1]:
empty_list.append(i)
print(empty_list)
def reverse_2(list_2):
empty_list = []
for i in range(len(list_2), 0, -1):
empty_list.append(list_2[i-1])
print(empty_list)
def reverse_3(list_2):
reversed_list = list(reversed(list_2))
print(reversed_list)
def reverse_4(list_2):
print(list_2[::-1])
#교수님의 사용방식
def reverse_5(list_2):
new_list = []
for i in list_2:
new_list.insert(0, i)
print(new_list)
#반장님의 아이디어
def reverse_6(list_2):
for i in range(len(list_2)):
list_2.insert(i, list_.pop())
print(list_2)
list_1 = [1, 2, 3, 4]
reverse(list_1)
reverse_2(list_1)
reverse_3(list_1)
reverse_4(list_1)
정말 다양한 방법이 있었는데 교수님이 이제 그냥 reversed 쓰라고 했다.
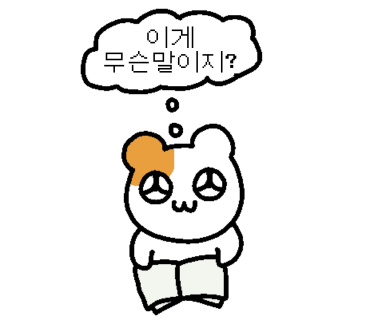
개발완료보고서
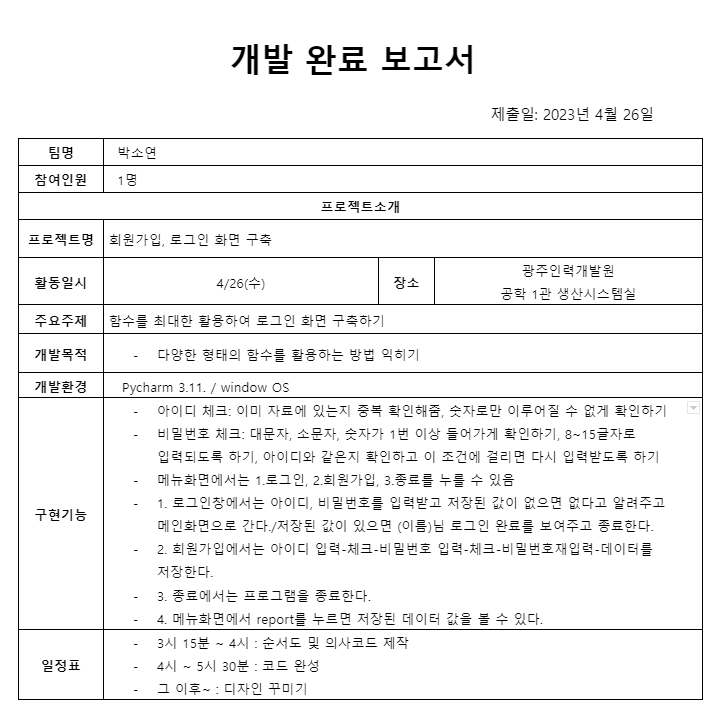
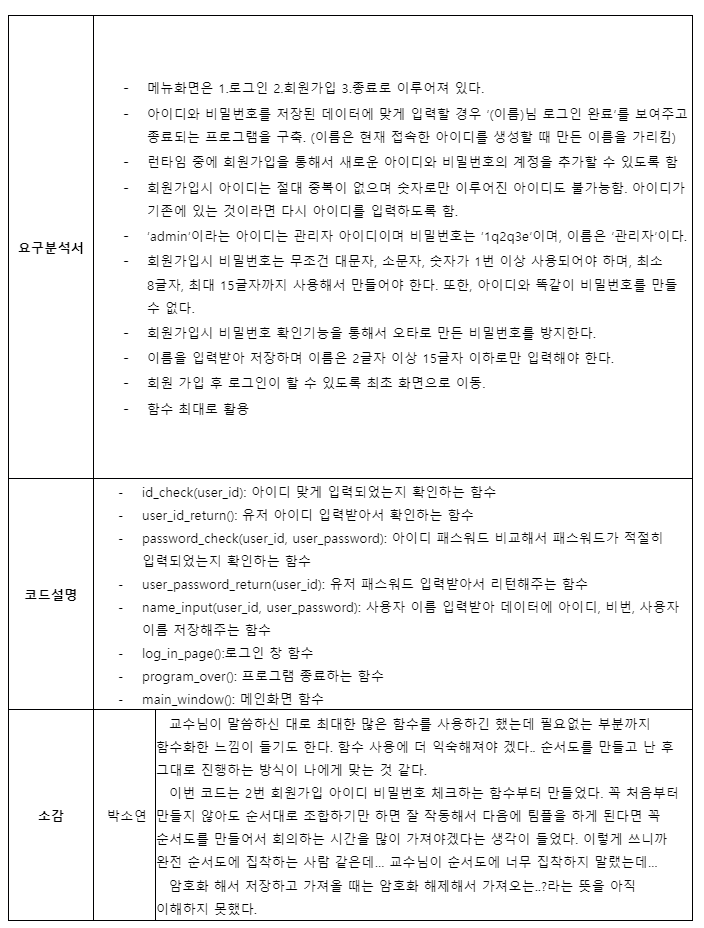
순서도
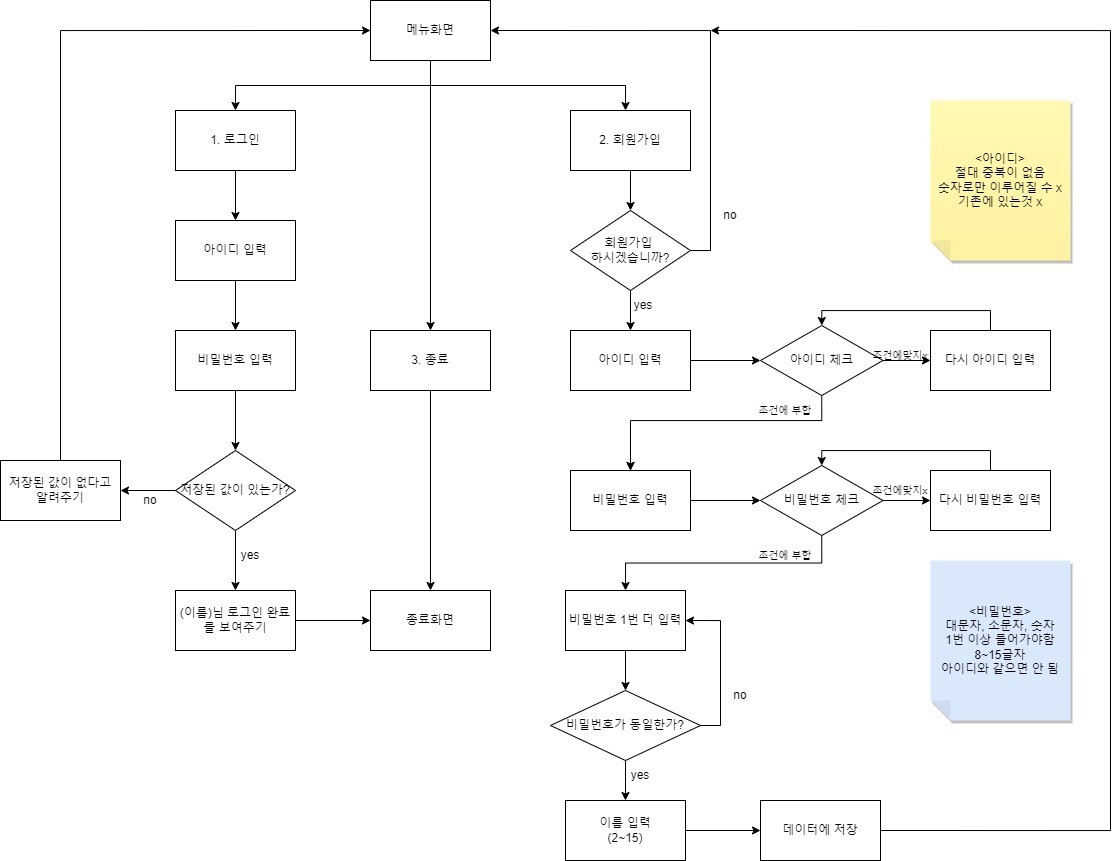
코드
1차 코드(디자인 전)
'''아이디 저장되는 데이터'''
data = {'관리자': ['admin', '1q2q3e']}
def id_check(user_id):
"""아이디 체크하는 함수"""
if user_id.isdigit():
print("숫자로만 이루어질 수 없습니다.")
return False
for i in data:
if data[i][0] == user_id:
print("기존에 존재하는 아이디입니다.")
return False
else:
return True
def user_id_return():
"""아이디 입력받아서 확인하는 함수"""
while True:
my_id = input("아이디를 입력해주세요: ")
if id_check(my_id):
break
return my_id
def password_check(user_id, user_password):
"""비밀번호 체크하는 함수"""
num, upper, lower = 0, 0, 0
# 글자가 8이상 15 이하여야 함
if not 8 <= len(user_password) <= 15:
print("8~15글자로 입력하세요!")
return False
if user_id == user_password:
print("아이디와 같은 비밀번호는 입력할 수 없습니다.")
return False
# 대문자, 소문자, 숫자 1번 이상 들어가야 함
for j in user_password:
if j.isdigit():
num += 1
if j.isupper():
upper += 1
if j.islower():
lower += 1
if num == 0:
print("숫자가 1개 이상 들어가야 합니다.")
return False
if upper == 0:
print("대문자가 1개 이상 들어가야 합니다.")
return False
if lower == 0:
print("소문자가 1개 이상 들어가야 합니다.")
return False
return user_password
def user_password_return(user_id):
"""패스워드 리턴해주는 함수"""
while True:
my_password = input("비밀번호를 입력하세요: ")
if password_check(user_id,my_password):
break
while True:
re_input_password = input("비밀번호를 다시 입력하세요: ")
if re_input_password == my_password:
return my_password
else:
print("두번째 입력한 비밀번호가 일치하지 않습니다! 다시 입력하세요.")
return my_password
def name_input(user_id, user_password):
"""사용자 이름 입력 함수"""
user_name = input("사용자의 이름을 입력하세요: ")
data[user_name] = [user_id, user_password]
print('정상적으로 저장되었습니다.')
print(f"사용자 이름:{user_name} 아이디:{user_id} 비밀번호:{len(user_password)*'*'}")
return main_window()
def log_in_page():
"""로그인 창 함수"""
print("여기는 로그인 창입니다.")
user_id = input("아이디를 입력하세요: ")
user_password = input("비밀번호를 입력하세요: ")
for i in data:
if data[i][0] == user_id and data[i][1] == user_password:
print(f"{i}님 로그인 되었습니다.")
program_over()
else:
print("저장된 내용이 없습니다. 회원가입하세요.")
def program_over():
exit("프로그램이 종료됩니다. ")
def main_window():
while True:
print("여기는 메인화면 입니다.")
print("1. 로그인 2.회원가입 3.종료")
user_choice = input("원하는 메뉴를 선택하세요: ")
if user_choice == '1':
log_in_page()
elif user_choice == '2':
my_id = user_id_return() # 아이디 받아오기
name_input(my_id, user_password_return(my_id)) # 사용자 입력
elif user_choice == '3':
program_over()
elif user_choice == 'report': #report를 누르면
print(data)
main_window()
2차 코드(디자인수정후)
import os
from console_art import login_art_top,login_art_middle, login_art_bottom, membership_register_middle, main_art
'''아이디 저장되는 데이터'''
data = {'관리자': ['admin', '1q2q3e']}
def id_check(user_id):
"""아이디 체크하는 함수"""
if user_id.isdigit():
print(f"{' '*4}>> ( • ㅁ•)!!숫자로만 이루어질 수 없습니다.")
return False
for i in data:
if data[i][0] == user_id:
print(f"{' '*4}>> ( • ㅁ•)!!기존에 존재하는 아이디입니다.")
return False
else:
return True
def user_id_return():
"""아이디 입력받아서 확인하는 함수"""
os.system("cls")
print(login_art_top + membership_register_middle + login_art_bottom)
while True:
my_id = input(f"{' '*4}>> 아이디를 입력해주세요: ")
if id_check(my_id):
break
return my_id
def password_check(user_id, user_password):
"""비밀번호 체크하는 함수"""
num, upper, lower = 0, 0, 0
# 글자가 8이상 15 이하여야 함
if not 8 <= len(user_password) <= 15:
print(f"{' '*4}>> ( • ▵•)!! 8~15글자로 입력하세요!")
return False
if user_id == user_password:
print(f"{' '*4}>> ( • ▵•)!! 아이디와 같은 비밀번호는 입력할 수 없습니다.")
return False
# 대문자, 소문자, 숫자 1번 이상 들어가야 함
for j in user_password:
if j.isdigit():
num += 1
if j.isupper():
upper += 1
if j.islower():
lower += 1
if num == 0:
print(f"{' '*4}>> ( • ㅁ•)!! 숫자가 1개 이상 들어가야 합니다.")
return False
if upper == 0:
print(f"{' '*4}>> ( • ㅁ•)!! 대문자가 1개 이상 들어가야 합니다.")
return False
if lower == 0:
print(f"{' '*4}>> ( • ㅁ•)!! 소문자가 1개 이상 들어가야 합니다.")
return False
return user_password
def user_password_return(user_id):
"""패스워드 리턴해주는 함수"""
while True:
my_password = input(f"{' '*4}>> 비밀번호를 입력하세요: ")
if password_check(user_id,my_password):
break
while True:
re_input_password = input(f"{' '*4}>> 비밀번호를 다시 입력하세요: ")
if re_input_password == my_password:
return my_password
else:
print(f"{' '*4}>> ( • ㅁ•)!! 두번째 입력한 비밀번호가 일치하지 않습니다! 다시 입력하세요.")
return my_password
def name_input(user_id, user_password):
"""사용자 이름 입력 함수"""
user_name = input(f"{' '*4}>> 사용자의 이름을 입력하세요: ")
data[user_name] = [user_id, user_password]
os.system('cls')
print(f"{login_art_top}{membership_register_middle}\n{' '*4}{' ̄'*32}")
print(f"{' '*4}| 정상적으로 저장되었습니다.(^ 0 ^){' '*27}|")
print(f"{' '*4}| 사용자 이름: {user_name} 아이디: {user_id} 비밀번호: {len(user_password)*'*':<15}{' '*4}|")
print(f"{' ' * 4}{' ̄' * 32}")
input(f"{' ' * 4}>> 메인화면으로 이동(enter) ")
# return main_window()
def log_in_page():
"""로그인 창 함수"""
os.system("cls")
print(login_art_top+login_art_middle+login_art_bottom)
user_id = input(f"{' '*4}>> 아이디를 입력하세요: ")
user_password = input(f"{' '*4}>> 비밀번호를 입력하세요: ")
for value in data.values():
if value[0] == user_id and value[1] == user_password:
os.system("cls")
print(login_art_top + login_art_middle)
print(f"{' ' * 4}{' ̄' * 32}")
print(f"{' ' * 4}|{' ' * 64}|")
print(f"{' ' * 4}| {value[0]:>8}님 로그인 되었습니다 ∩(^ 0 ^)∩ {' ' * 21}|")
print(f"{' ' * 4}| 목요일 메뉴는 닭곰탕, 가자미무조림, 꽃맞살어묵볶음입니다. |")
print(f"{' ' * 4}{' ̄' * 32}")
input(f"{' ' * 4}>> 종료(enter) ")
os.system("cls")
program_over()
print(f"{' '*4}!! 저장된 내용이 없습니다. 회원가입하세요.")
input(f"{' '*6} 회원가입하러가기(enter)>")
os.system('cls')
def program_over():
os.system("cls")
exit(f"{login_art_top}\n"
f"{' '*4}| 프로그램을 종료합니다.{' '*39}|\n"
f"{' ' * 4}|{' ' * 64}|\n"
f"{' '*4}{' ̄'*32}")
def main_window():
os.system("cls")
while True:
os.system("cls")
print(main_art)
user_choice = input(f"{' '*4}원하는 메뉴를 선택하세요 : ")
if user_choice == '1':
log_in_page()
elif user_choice == '2':
my_id = user_id_return() # 아이디 받아오기
name_input(my_id, user_password_return(my_id)) # 사용자 입력
elif user_choice == '3':
program_over()
main_window()
console_art.py
login_art_top = f"""
{' ̄'*32}"""
login_art_middle = f"""
| 로그인 창 [-][口][×] |"""
membership_register_middle = """
| 회원가입창 [-][口][×] |"""
login_art_bottom = f"""
{" ̄"*32}
|{" "*64}|
| ______ _______ |
| ID : Password: |
|  ̄ ̄ ̄ ̄ ̄ ̄  ̄ ̄ ̄ ̄ ̄ ̄ ̄ |
{" ̄"*32} """
main_art = """
 ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄
| 메인화면 [-][口][×] |
| ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄|
| 광주인력개발원 KDT AI 3회차 과정에 오신것을 환영합니다!! |
| 식단메뉴를 보고 싶으면 로그인하세요. |
| ______ ______ _____ |
| |1. 로그인 | |2. 회원가입| | 3.종료 | |
|  ̄ ̄ ̄ ̄ ̄ ̄  ̄ ̄ ̄ ̄ ̄ ̄  ̄ ̄ ̄ ̄ ̄ |
 ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄
"""
코드구현사진
(디자인수정전)
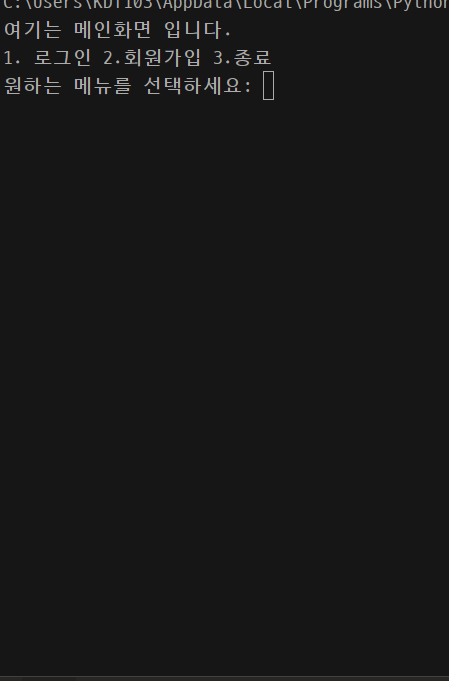
(디자인수정후)
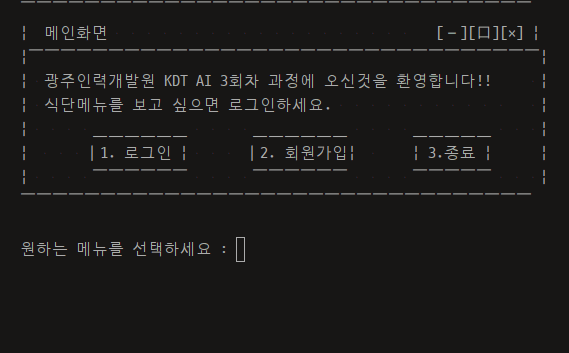
로그인하기
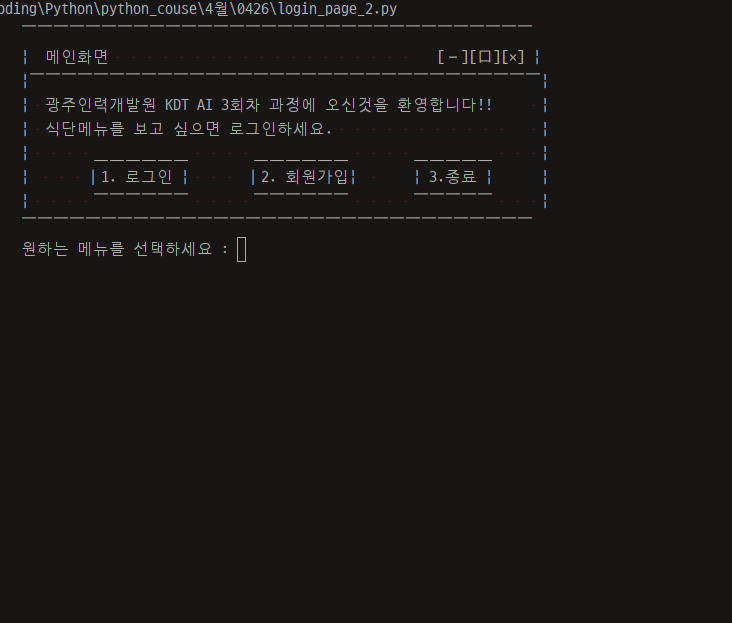
회원가입하기
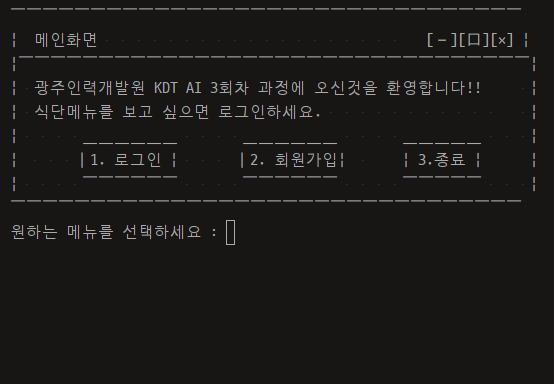
아이디 잘못 입력했을 때
만난 오류 및 해결방안
1. data 딕셔너리에 아이디 비밀번호가 저장되지 않음 -> while문 안에 넣어서 해결완.
더 찾아보니까 pickle()모듈을 사용해 객체를 파일로 저장하고 불러올 수 있다고 한다.
2. for문 내 띄어쓰기 문제
문제의 코드는 이 함수였는데, if문이 실행되지 않고 else문이 실행된 후 ->
다시 if문이 실행되는게 문제였다.
그래서 파이썬 튜터로 돌려본 후 나의 바보같은 else위치를 깨달았다.
def log_in_page():
"""로그인 창 함수"""
user_id = input("아이디를 입력하세요: ")
user_password = input(" 비밀번호를 입력하세요: ")
for value in data.values():
if value[0] == user_id and value[1] == user_password:
os.system("cls")
print(f"{' ' * 4}| {value[0]:>8}님 로그인 되었습니다")
input(f"{' ' * 4}>> 종료(enter) ")
os.system("cls")
program_over()
else:
print(f"저장된 내용이 없습니다. 회원가입하세요.")
바로 else문을 for문에 넣어줄 필요가 없었던 것이다...&&^^
내가 원하는 건 for문을 완전히 돌려준 후 if문을 만족하면 실행시키고 그렇지 않으면 else문을 실행시키는 것이었는데 그럼 당연히 for문 안에 if문만 들어갔어야 했다. ..
수정후 코드
def log_in_page():
"""로그인 창 함수"""
user_id = input("아이디를 입력하세요: ")
user_password = input(" 비밀번호를 입력하세요: ")
for value in data.values():
if value[0] == user_id and value[1] == user_password:
os.system("cls")
print(f"{' ' * 4}| {value[0]:>8}님 로그인 되었습니다")
input(f"{' ' * 4}>> 종료(enter) ")
os.system("cls")
program_over()
print(f"저장된 내용이 없습니다. 회원가입하세요.")
else의 필요성이 없으므로 지워주고 들여쓰기를 삭제해줬다.
오늘 새로 배운 내용
isinstance 함수(내장함수)
파이썬에는 기본으로 제공되는 여러 가지 함수들 중 하나이며, 이 함수는 import 없이 바로 사용할 수 있다.
이 함수는 첫 번째 인자가 두 번째 인자의 인스턴스인지 확인하는 함수이다.
예를 들어, 이렇게 사용할 수 있다.
x = 5
print(isinstance(x, int)) # True
print(isinstance(x, str)) # False
키워드 매개변수
키워드 매개변수는 기본값이 있는 매개변수를 사용하는 것으로, 함수를 호출할 때 매개변수 이름을 지정하여 값을 전달할 수 있다. 예를 들어,
def greet(name, greeting="Hello"):
print(f"{greeting}, {name}!")
이 함수는 name 매개변수와 기본값이 "Hello"인 greeting 매개변수를 가지고 있다. greet함수를 호출할 때, greeting 매개변수의 값을 지정하지 않으면 기본값인 'Hello'가 사용된다.
주의: 함수의 이름과 같은 변수명 만들지 말기
++ 암호화 복호화 부분 추가
아스키 코드 숫자를 활용해,
1부터 10까지 랜덤으로 하나의 숫자를 반환해 사용자의 비밀번호를 암호화 해서 넣는 함수를 만들었다.
이런식으로 암호화되서 저장된다.
그리고 로그인할때는 사용자가 입력한 아이디가 일치하면 이동숫자만큼 암호를 다시 복호화하고,
일치하면 로그인을 해준다.
import random
'''아이디 저장되는 데이터'''
data = {'관리자': ['admin', '1q2q3e', 0]}
def id_check(user_id):
"""아이디 체크하는 함수"""
if user_id.isdigit():
print("숫자로만 이루어질 수 없습니다.")
return False
for i in data:
if data[i][0] == user_id:
print("기존에 존재하는 아이디입니다.")
return False
else:
return True
def user_id_return():
"""아이디 입력받아서 확인하는 함수"""
while True:
my_id = input("아이디를 입력해주세요: ")
if id_check(my_id):
break
return my_id
def password_check(user_id, user_password):
"""비밀번호 체크하는 함수"""
num, upper, lower = 0, 0, 0
# 글자가 8이상 15 이하여야 함
if not 8 <= len(user_password) <= 15:
print("8~15글자로 입력하세요!")
return False
if user_id == user_password:
print("아이디와 같은 비밀번호는 입력할 수 없습니다.")
return False
# 대문자, 소문자, 숫자 1번 이상 들어가야 함
for j in user_password:
if j.isdigit():
num += 1
if j.isupper():
upper += 1
if j.islower():
lower += 1
if num == 0:
print("숫자가 1개 이상 들어가야 합니다.")
return False
if upper == 0:
print("대문자가 1개 이상 들어가야 합니다.")
return False
if lower == 0:
print("소문자가 1개 이상 들어가야 합니다.")
return False
return user_password
def user_password_return(user_id):
"""패스워드 리턴해주는 함수"""
while True:
my_password = input("비밀번호를 입력하세요: ")
if password_check(user_id,my_password):
break
while True:
re_input_password = input("비밀번호를 다시 입력하세요: ")
if re_input_password == my_password:
return encoding_password(my_password)
else:
print("두번째 입력한 비밀번호가 일치하지 않습니다! 다시 입력하세요.")
def encoding_password(user_password):
"""패스워드 암호화해주는 함수"""
new_password_list = []
move_cnt = random.randint(1, 10)
for i in user_password:
new_password_list.append(chr(ord(i) - move_cnt))
new_passowrd = ''.join(new_password_list)
return new_passowrd, move_cnt
def decoding_password(encoded_password, mv_cnt):
"""패스워드 복호화하는 함수"""
new_password_list = []
for i in encoded_password:
new_password_list.append(chr(ord(i) + mv_cnt))
decoded_password = ''.join(new_password_list)
return decoded_password
def name_input(user_id, user_password, move_cnt):
"""사용자 이름 입력 함수"""
while True:
user_name = input("사용자의 이름을 입력하세요: ")
if not 2 <= len(user_name) <=15:
print("2 ~ 15의 숫자로만 입력할 수 있습니다.")
else:
break
data[user_name] = [user_id, user_password, move_cnt]
print('정상적으로 저장되었습니다.')
print(f"사용자 이름:{user_name} 아이디:{user_id} 비밀번호:{len(user_password)*'*'}")
return main_window()
def log_in_page():
"""로그인 창 함수"""
print("여기는 로그인 창 입니다.")
user_id = input("아이디를 입력하세요: ")
user_password = input("비밀번호를 입력하세요: ")
for i, value in enumerate(data.values()):
if value[0] == user_id:
decoded_password = decoding_password(value[1], value[2])
if user_password == decoded_password:
print(f"{list(data.keys())[i]}님 로그인에 성공했습니다.")
program_over()
print("저장된 내용이 없습니다. 회원가입하세요.")
def program_over():
exit("프로그램이 종료됩니다. ")
def report():
for i in data:
print(f"이름 : {i:^15} | 아이디 : {data[i][0]:^8} | 비밀번호 : {data[i][1]:^15} | 이동 숫자 : {data[i][2]}")
def main_window():
while True:
print("여기는 메인화면 입니다.")
print("1. 로그인 2.회원가입 3.종료")
user_choice = input("원하는 메뉴를 선택하세요: ")
if user_choice == '1':
log_in_page()
elif user_choice == '2':
my_id = user_id_return() # 아이디 받아오기
encoded_password, move_cnt = user_password_return(my_id)
name_input(my_id,encoded_password, move_cnt) # 사용자 입력
elif user_choice == '3':
program_over()
elif user_choice == 'report':
report()
main_window()
'개발공부 > Python' 카테고리의 다른 글
[파이썬] 추억의 게임 고향만두 텍스트 게임으로 만들기(반복문, 조건문) (0) | 2023.04.29 |
---|---|
[파이썬] 간단한 버스 정류장 조회 시스템 만들기 (0) | 2023.04.29 |
[파이썬] 개복치 키우는 게임 만들기 (0) | 2023.04.26 |
[파이썬] 숫자야구 함수화해서 만들기 (0) | 2023.04.26 |
[파이썬] 60갑자 조합 만들어보기 (0) | 2023.04.24 |