간단한 단어조합표 만들기
1. 코드
<python />
import sys
from PyQt5.QtWidgets import *
class Window(QWidget):
def __init__(self):
super().__init__()
self.button1 = QPushButton("안녕하세요!")
self.button1.setFixedSize(150, 60)
self.button1.setStyleSheet(
"color: white;"
"background-color:rgb(235, 83, 83);"
"border-radius:5px;"
)
self.button1.clicked.connect(self.onClick)
self.button2 = QPushButton("저는 지금")
self.button2.setFixedSize(150, 60)
self.button2.setStyleSheet(
"color: black;"
"background-color:rgb(249, 217, 35);"
"border-radius:5px;"
)
self.button2.clicked.connect(self.onClick2)
self.button3 = QPushButton("파이큐티 공부를")
self.button3.setFixedSize(150, 60)
self.button3.setStyleSheet(
"color: white;"
"background-color:rgb(54, 174, 124);"
"border-radius:5px;"
)
self.button3.clicked.connect(self.onClick3)
self.button4 = QPushButton("할 것입니다.")
self.button4.setFixedSize(150, 60)
self.button4.setStyleSheet(
"color: white;"
"background-color:rgb(24, 116, 152);"
"border-radius:5px;"
)
self.button4.clicked.connect(self.onClick4)
self.button5 = QPushButton("내일은 어린이날!")
self.button5.setFixedSize(150, 60)
self.button5.setStyleSheet(
"color: white;"
"background-color:rgb(109, 169, 228);"
"border-radius:5px;"
)
self.button5.clicked.connect(self.onClick5)
self.button6 = QPushButton("공부를 합시다.")
self.button6.setFixedSize(150, 60)
self.button6.setStyleSheet(
"color: black;"
"background-color:rgb(173, 228, 219);"
"border-radius:5px;"
)
self.button6.clicked.connect(self.onClick6)
self.label = QTextEdit()
self.label.setFixedSize(460, 40)
self.button7 = QPushButton("확인")
self.button7.setFixedSize(460,25)
self.button7.setStyleSheet(
"color: white;"
"background-color:rgb(58, 134, 255);"
"border-radius:5px;"
)
self.button7.clicked.connect(self.onclick7)
main_layout = QGridLayout()
main_layout.addWidget(self.button1, 0, 0)
main_layout.addWidget(self.button2, 0, 1)
main_layout.addWidget(self.button3, 0, 2)
main_layout.addWidget(self.button4, 1, 0)
main_layout.addWidget(self.button5, 1, 1)
main_layout.addWidget(self.button6, 1, 2)
layout = QVBoxLayout()
layout.addLayout(main_layout)
layout.addWidget(self.label)
layout.addWidget(self.button7)
self.setLayout(layout)
self.setWindowTitle("단어조합표")
def onClick(self):
text = self.button1.text()
if text == "안녕하세요!":
self.button1.setText("안녕하지 못합니다.")
else:
self.button1.setText("안녕하세요!")
def onClick2(self):
text = self.button2.text()
if text == "저는 지금":
self.button2.setText("저는 저녁을 먹고")
else:
self.button2.setText("저는 지금")
def onClick3(self):
text = self.button3.text()
if text == "파이큐티 공부를":
self.button3.setText("클래스 공부를")
else:
self.button3.setText("파이큐티 공부를")
def onClick4(self):
text = self.button4.text()
if text == "할 것입니다.":
self.button4.setText("안할건데요 루꾸루삥뽕")
else:
self.button4.setText("할 것입니다.")
def onClick5(self):
text = self.button5.text()
if text == "내일은 어린이날!":
self.button5.setText("내일은..어른이날..")
else:
self.button5.setText("내일은 어린이날!")
def onClick6(self):
text = self.button6.text()
if text == "공부를 합시다.":
self.button6.setText("해야겠죠..? 공부..?")
else:
self.button6.setText("공부를 합시다.")
def onclick7(self):
text = self.button1.text()
text2 = self.button2.text()
text3 = self.button3.text()
text4 = self.button4.text()
text5 = self.button5.text()
text6 = self.button6.text()
self.label.setText(f"{text} {text2} {text3} {text4} {text5} {text6}")
if __name__ == "__main__":
app = QApplication(sys.argv)
window = Window()
window.show()
sys.exit(app.exec())
2. 실행결과
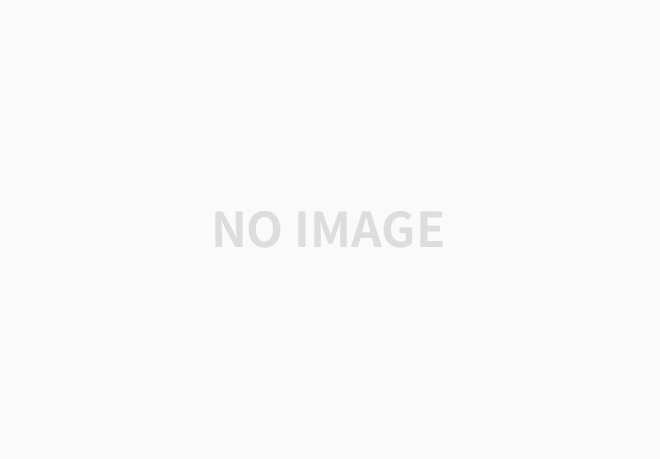
색상을 넣어서 좀 화사하게 만들어 봤다.
3. 참고 사이트
색상조합: https://colorhunt.co/
Color Palettes for Designers and Artists - Color Hunt
Discover the newest hand-picked color palettes of Color Hunt. Get color inspiration for your design and art projects.
colorhunt.co
푸시버튼 공부: https://www.pythonguis.com/docs/qpushbutton/
PyQt QPushButton — A universal Button Widget
QPushButton is a clickable widget which you can use to trigger actions in your UI. The push button, or command button, is perhaps the most commonly used widget in any graphical user interface (GUI).
www.pythonguis.com
푸시버튼 css 참조 블로그: https://life-of-panda.tistory.com/72
[Qt/PyQt] QPushButton CSS StyleSheet 예제 모음
사용한 색상표입니다. rgb(255, 190, 11) rgb(251, 86, 7) rgb(255, 0, 110) rgb(131, 56, 236) rgb(58, 134, 255) QPushButton{ color: white; background-color: rgb(58, 134, 255); border-radius: 5px; } QPushButton{ color: rgb(58, 134, 255); background-col
life-of-panda.tistory.com
'개발공부 > Pyqt 파이큐티' 카테고리의 다른 글
[PyQt] 파이큐티와 qt designer활용해 행맨 만들기 (0) | 2023.05.05 |
---|---|
[PyQt] 파이큐티 콘솔창에서 다운로드하기 (0) | 2023.05.04 |
[파이큐티] 허접한 가위바위보 게임 만들기 (0) | 2023.05.04 |
[파이큐티] 클릭시 버튼 이름 연결하기(.sender(), .text()) (1) | 2023.05.04 |
[파이큐티] qt designer 사용하기 (0) | 2023.05.03 |