콤보박스, 체크박스, 푸시버튼 3가지 종류를 사용하여 로또 만들어보기
폰트: G마켓 산스
qt designer로 만든 화면
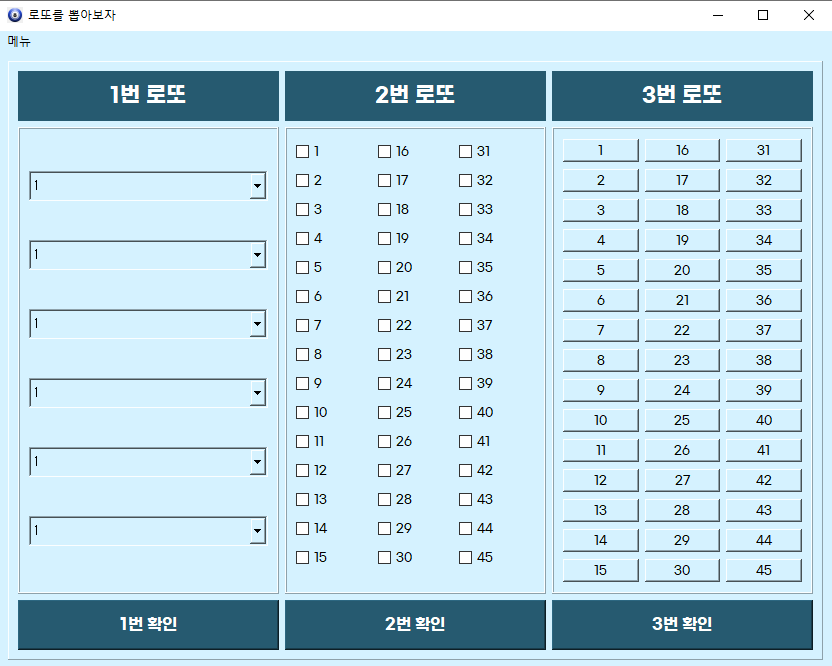
코드
import os
import sys
import random
from PyQt5.QtWidgets import *
from PyQt5 import uic
from PyQt5.QtGui import *
from PyQt5.QtCore import *
def resource_path(relative_path):
base_path = getattr(sys, "_MEIPASS", os.path.dirname(os.path.abspath(__file__)))
return os.path.join(base_path, relative_path)
form = resource_path('lotto.ui')
form_class = uic.loadUiType(form)[0]
class WindowClass(QMainWindow, form_class):
def __init__(self):
super( ).__init__( )
self.setupUi(self)
# 콤보박스에 숫자 넣기
self.combobox_list = [self.comboBox, self.comboBox_2, self.comboBox_3, self.comboBox_4, self.comboBox_5, self.comboBox_6]
for i in self.combobox_list:
i.addItems([str(e) for e in range(1, 46)])
# 콤보박스 확인 눌렀을 때 랜덤 숫자 확인
self.lotto_1_check.clicked.connect(self.combobox_cnt_check)
#체크박스 리스트
self.checkbox_list = [
self.checkBox_1,
self.checkBox_2,
self.checkBox_3,
self.checkBox_4,
self.checkBox_5,
self.checkBox_6,
self.checkBox_7,
self.checkBox_8,
self.checkBox_9,
self.checkBox_10,
self.checkBox_11,
self.checkBox_12,
self.checkBox_13,
self.checkBox_14,
self.checkBox_15,
self.checkBox_16,
self.checkBox_17,
self.checkBox_18,
self.checkBox_19,
self.checkBox_20,
self.checkBox_21,
self.checkBox_22,
self.checkBox_23,
self.checkBox_24,
self.checkBox_25,
self.checkBox_26,
self.checkBox_27,
self.checkBox_28,
self.checkBox_29,
self.checkBox_30,
self.checkBox_31,
self.checkBox_32,
self.checkBox_33,
self.checkBox_34,
self.checkBox_35,
self.checkBox_36,
self.checkBox_37,
self.checkBox_38,
self.checkBox_39,
self.checkBox_40,
self.checkBox_41,
self.checkBox_42,
self.checkBox_43,
self.checkBox_44,
self.checkBox_45,
]
#체크박스 확인 눌렀을 때 랜덤 숫자 확인
self.lotto_2_check.clicked.connect(self.checkbox_cnt_check)
#푸시버튼 리스트
self.pushButton_list = [
self.pushButton_1,
self.pushButton_2,
self.pushButton_3,
self.pushButton_4,
self.pushButton_5,
self.pushButton_6,
self.pushButton_7,
self.pushButton_8,
self.pushButton_9,
self.pushButton_10,
self.pushButton_11,
self.pushButton_12,
self.pushButton_13,
self.pushButton_14,
self.pushButton_15,
self.pushButton_16,
self.pushButton_17,
self.pushButton_18,
self.pushButton_19,
self.pushButton_20,
self.pushButton_21,
self.pushButton_22,
self.pushButton_23,
self.pushButton_24,
self.pushButton_25,
self.pushButton_26,
self.pushButton_27,
self.pushButton_28,
self.pushButton_29,
self.pushButton_30,
self.pushButton_31,
self.pushButton_32,
self.pushButton_33,
self.pushButton_34,
self.pushButton_35,
self.pushButton_36,
self.pushButton_37,
self.pushButton_38,
self.pushButton_39,
self.pushButton_40,
self.pushButton_41,
self.pushButton_42,
self.pushButton_43,
self.pushButton_44,
self.pushButton_45,]
#푸시버튼 확인 눌렀을 때 랜덤 숫자 확인
self.lotto_3_check.clicked.connect(self.pushbutton_cnt_check)
#메뉴바 QAction 연결
self.action_exit.triggered.connect(QCoreApplication.instance().quit)
self.action_replay.triggered.connect(self.replay)
# self.setStyleSheet("action_replay :hover {background-color:black}")
# self.setStyleSheet("QToolButton:hover {background-color:blue} QToolButton:!hover { background-color: lightgray }")
def exit_code(self):
print("끕니다.")
def replay(self):
for i in self.checkbox_list:
i.setStyleSheet("background-color: rgb(213, 242, 255)")
if i.isChecked():
i.setChecked(False)
for j in self.combobox_list:
j.clear() #이 방법밖에 없는걸까?
for i in self.combobox_list:
i.addItems([str(e) for e in range(1, 46)])
for k in self.pushButton_list:
k.setStyleSheet("background-color: rgb(213, 242, 255)")
k.setChecked(False)
def combobox_cnt_check(self):
cnt, cnt_2 = 0, 0
reply = ''
random_num = [str(num) for num in random.sample(range(1, 45), 7)] #랜덤 숫자 뽑아서 str형으로 변환
user_guess = []
for i in self.combobox_list: #사용자가 유추한 숫자 리스트에 담기
user_guess.append(i.currentText())
print(user_guess)
print(random_num[:6])
print(random_num[6])
for j in user_guess:
if j in random_num[:6]:
cnt += 1
if j == random_num[6]:
cnt_2 += 1
if cnt == 6:
reply += "1등입니다."
elif cnt == 5 and cnt_2 == 1:
reply += "2등입니다."
elif cnt == 4:
reply += "3등입니다."
elif cnt == 3:
reply += "5등입니다.."
else:
reply += "아쉽게도 당첨되지 않았습니다. 다음 기회에 도전하세요!\n"
reply += f"당첨 번호는 {','.join(random_num)}입니다."
num_1_answer = QMessageBox()
num_1_answer.setText(reply)
num_1_answer.exec_()
def checkbox_cnt_check(self):
"""체크한 갯수가 6개인지 확인및 랜덤숫자체크"""
random_num = [str(num) for num in random.sample(range(1, 45), 7)]
print(random_num)
cnt = 0
cnt_2 = 0
text = ''
checked_box = 0
for checkbox in self.checkbox_list:
if checkbox.isChecked():
checked_box += 1
if checkbox.text() in random_num[:7]:
cnt += 1
if checked_box > 6 or checked_box < 6:
reply = QMessageBox()
reply.setText("6개의 버튼을 선택하세요")
reply.exec_()
else:
for i in self.checkbox_list:
# check_num += i.text()
if i.text() in random_num:
i.setStyleSheet("background-color: yellow")
# if i.isChecked():
# cnt += 1
else:
i.setStyleSheet("")
if cnt == 6:
text += "축하합니다! 1등에 당첨되셨습니다."
elif cnt == 5:
for i in self.checkbox_list:
if i.text() in str(random_num[6:]):
cnt_2 += 1
if cnt_2 == 1:
text += "축하합니다! 2등에 당첨되셨습니다."
else:
text += "축하합니다! 3등에 당첨되셨습니다."
elif cnt == 4:
text += "축하합니다! 4등에 당첨되셨습니다."
elif cnt == 3:
text += "축하합니다! 5등에 당첨되셨습니다."
else:
text += "아쉽게도 당첨되지 않았습니다. 다음 기회에 도전해보세요!"
num_2_message = QMessageBox()
num_2_message.setText(f"{text}\n당첨번호는 {','.join(random_num)}입니다.")
num_2_message.exec_()
def pushbutton_cnt_check(self):
random_num = [str(num) for num in random.sample(range(1, 45), 7)]
checked_btn = []
cnt, cnt_2 = 0, 0
text =''
for i in self.pushButton_list:
if i.text() in random_num:
i.setStyleSheet("background-color: yellow")
if i.isChecked():
a = i.text()
checked_btn.append(a)
for j in random_num[:6]:
if j in checked_btn:
cnt += 1
if random_num[6] in checked_btn:
cnt_2 += 1
print(checked_btn) #확인용
if cnt == 6:
text += "축하합니다! 1등에 당첨되셨습니다."
elif cnt == 5 and cnt_2 == 1:
text += "축하합니다! 2등에 당첨되셨습니다."
elif cnt == 5:
text += "축하합니다! 3등에 당첨되셨습니다."
elif cnt == 4:
text += "축하합니다! 4등에 당첨되셨습니다."
elif cnt == 3:
text += "축하합니다! 5등에 당첨되셨습니다."
else:
text += "아쉽게도 당첨되지 않았습니다. 다음 기회에 도전해보세요!"
num_2_message = QMessageBox()
num_2_message.setText(f"{text}\n당첨번호는 {','.join(random_num)}입니다.")
num_2_message.exec_()
if __name__ == '__main__':
app = QApplication(sys.argv)
myWindow = WindowClass( )
myWindow.show( )
app.exec_( )
코드설명
# 콤보박스에 숫자 넣기
self.combobox_list = [self.comboBox, self.comboBox_2, self.comboBox_3, self.comboBox_4, self.comboBox_5, self.comboBox_6]
for i in self.combobox_list:
i.addItems([str(e) for e in range(1, 46)])
# 콤보박스 확인 눌렀을 때 랜덤 숫자 확인
self.lotto_1_check.clicked.connect(self.combobox_cnt_check)
콤보박스 리스트 만들어서 변수명 선언한 후
각각의 리스트에 1부터 45까지 넣어주기
그리고 콤보박스 확인 버튼 눌렀을 때 combobox_cnt_check로 이동하도록 한다.
combobox_cnt_check 함수
def combobox_cnt_check(self):
cnt, cnt_2 = 0, 0
reply = ''
random_num = [str(num) for num in random.sample(range(1, 45), 7)] #랜덤 숫자 뽑아서 str형으로 변환
user_guess = []
for i in self.combobox_list: #사용자가 유추한 숫자 리스트에 담기
user_guess.append(i.currentText())
print(user_guess)
print(random_num[:6])
print(random_num[6])
for j in user_guess:
if j in random_num[:6]:
cnt += 1
if j == random_num[6]:
cnt_2 += 1
if cnt == 6:
reply += "1등입니다."
elif cnt == 5 and cnt_2 == 1:
reply += "2등입니다."
elif cnt == 4:
reply += "3등입니다."
elif cnt == 3:
reply += "5등입니다.."
else:
reply += "아쉽게도 당첨되지 않았습니다. 다음 기회에 도전하세요!\n"
reply += f"당첨 번호는 {','.join(random_num)}입니다."
num_1_answer = QMessageBox()
num_1_answer.setText(reply)
num_1_answer.exec_()
랜덤 숫자 맞았는지 확인할 변수 cnt, 보너스 점수 맞았는지 확인할 변수 cnt_2를 0 으로 만들어주고
랜덤 숫자를 뽑아서 str로 변환해준다.
사용자가 유추한 숫자를 currentText()를 사용해서 user_guess라는 빈 리스트에 담아준다.
사용자가 유추한 숫자 user_guess를 for문으로 돌려서
만약 1번부터 6번까지 맞았다면 cnt += 1을, 보너스 숫자도 맞았으면 cnt_2 += 1을 해 준다.
cnt가 6이면 빈 문자열 reply 에 1등입니다. 라고 더해주기
cnt가 5이고 cnt_2가 1이면 reply에 2등입니다. 라고 더해주기
cnt가 4이면 빈 문자열 reply 에 3등입니다. 라고 더해주기
cnt가 3이면 빈 문자열 reply 에 5등입니다. 라고 더해주기
아무것도 해당하지 않으면 당첨되지 않았다는 말을 더해주고
당첨 번호도 더해준 후
메세지박스를 만들어서 reply를 setText해준다.
#체크박스 리스트
self.checkbox_list = [
self.checkBox_1,
...(생략)...
self.checkBox_45,
]
#체크박스 확인 눌렀을 때 랜덤 숫자 확인
self.lotto_2_check.clicked.connect(self.checkbox_cnt_check)
qt designer에서 만들었던 체크박스 45개를 리스트에 넣어주고
2번 확인 버튼을 눌렀을 때 checkbox_cnt_check 함수로 넘어가도록 한다.
checkbox_cnt_check 함수
def checkbox_cnt_check(self):
"""체크한 갯수가 6개인지 확인및 랜덤숫자체크"""
random_num = [str(num) for num in random.sample(range(1, 45), 7)]
print(random_num)
cnt = 0
cnt_2 = 0
text = ''
checked_box = 0
for checkbox in self.checkbox_list:
if checkbox.isChecked():
checked_box += 1
if checkbox.text() in random_num[:7]:
cnt += 1
if checked_box > 6 or checked_box < 6:
reply = QMessageBox()
reply.setText("6개의 버튼을 선택하세요")
reply.exec_()
else:
for i in self.checkbox_list:
# check_num += i.text()
if i.text() in random_num:
i.setStyleSheet("background-color: yellow")
# if i.isChecked():
# cnt += 1
else:
i.setStyleSheet("")
if cnt == 6:
text += "축하합니다! 1등에 당첨되셨습니다."
elif cnt == 5:
for i in self.checkbox_list:
if i.text() in str(random_num[6:]):
cnt_2 += 1
if cnt_2 == 1:
text += "축하합니다! 2등에 당첨되셨습니다."
else:
text += "축하합니다! 3등에 당첨되셨습니다."
elif cnt == 4:
text += "축하합니다! 4등에 당첨되셨습니다."
elif cnt == 3:
text += "축하합니다! 5등에 당첨되셨습니다."
else:
text += "아쉽게도 당첨되지 않았습니다. 다음 기회에 도전해보세요!"
num_2_message = QMessageBox()
num_2_message.setText(f"{text}\n당첨번호는 {','.join(random_num)}입니다.")
num_2_message.exec_()
랜덤 숫자를 str로 담아주고
로또번호 6개, 보너스번호 1개가 담길 변수명 cnt, cnt_2를 만들어준다.
메세지박스에 담길 text와 예외처리를 위한 checked_box라는 빈 리스트를 만들었다.
체크된 갯수가 6개가 아니면 6개를 선택하라는 메세지를 출력한다.
체크된 갯수가 6개라면,
위에서 선언한 체크박스 리스트를 for문을 돌려서
체크박스의 text()를 가져와 랜덤으로 선택된 숫자와 비교한 후 맞으면 그 숫자 배경을 노란색으로 칠한다.
밑에 확인하는 부분은 위와 동일
#푸시버튼 리스트
self.pushButton_list = [
self.pushButton_1,
...(생략)...
self.pushButton_45,]
#푸시버튼 확인 눌렀을 때 랜덤 숫자 확인
self.lotto_3_check.clicked.connect(self.pushbutton_cnt_check)
qt designer에서 만들었던 푸시버튼 45개를 리스트에 넣어주고
2번 확인 버튼을 눌렀을 때 pushbutton_cnt_check 함수로 넘어가도록 한다.
pushbutton_cnt_check 함수
def pushbutton_cnt_check(self):
random_num = [str(num) for num in random.sample(range(1, 45), 7)]
checked_btn = []
cnt, cnt_2 = 0, 0
text =''
for i in self.pushButton_list:
if i.text() in random_num:
i.setStyleSheet("background-color: yellow")
if i.isChecked():
checked_btn.append(i.text())
for j in random_num[:6]:
if j in checked_btn:
cnt += 1
if random_num[6] in checked_btn:
cnt_2 += 1
# print(checked_btn) #확인용
if cnt == 6:
text += "축하합니다! 1등에 당첨되셨습니다."
elif cnt == 5 and cnt_2 == 1:
text += "축하합니다! 2등에 당첨되셨습니다."
elif cnt == 5:
text += "축하합니다! 3등에 당첨되셨습니다."
elif cnt == 4:
text += "축하합니다! 4등에 당첨되셨습니다."
elif cnt == 3:
text += "축하합니다! 5등에 당첨되셨습니다."
else:
text += "아쉽게도 당첨되지 않았습니다. 다음 기회에 도전해보세요!"
num_2_message = QMessageBox()
num_2_message.setText(f"{text}\n당첨번호는 {','.join(random_num)}입니다.")
num_2_message.exec_()
위 함수와 동일하게 랜덤 함수를 문자열로 만들어주고,
checked_btn이라는 빈 리스트를 만든다.
똑같이 번호체크용으로 cnt, cnt_2(보너스용) 만들어준다.
위에서 만들었던 버튼 리스트를 for문으로 돌려서
.text()로 객체의 이름을 가져와주고, 랜덤숫자와 동일하면 배경으로 노란색으로 칠해준다.
그리고 버튼이 눌렸다면(유저가 버튼을 선택하면) checked_btn에 담아준다.
랜덤숫자 6번까지 맞았는지 확인하고 있으면 cnt += 1
보너스 숫자 맞았는지 확인하고 있다면 cnt_2 += 1
밑에 메세지박스와 체크하는건 위 함수와 같다.
#메뉴바 QAction 연결
self.action_exit.triggered.connect(QCoreApplication.instance().quit)
self.action_replay.triggered.connect(self.replay)
# self.setStyleSheet("action_replay :hover {background-color:black}")
# self.setStyleSheet("QToolButton:hover {background-color:blue} QToolButton:!hover { background-color: lightgray }")
메뉴바 QAction ->qt designer에서 replay와 exit버튼을 만들었다.
그래서 각 버튼을 누르면 꺼지고 / replay 함수로 연결되게 했다.
replay 함수
def replay(self):
for i in self.checkbox_list:
i.setStyleSheet("background-color: rgb(213, 242, 255)")
if i.isChecked():
i.setChecked(False)
for j in self.combobox_list:
j.clear() #이 방법밖에 없는걸까?
for i in self.combobox_list:
i.addItems([str(e) for e in range(1, 46)])
for k in self.pushButton_list:
k.setStyleSheet("background-color: rgb(213, 242, 255)")
k.setChecked(False)
다시할지를 누르면
체크박스 리스트는 - 체크된 항목이 모두 False된다. 그리고 배경을 모두 기본값으로 변경한다.
콤보박스 리스트는 - 모두 clear() 하고 다시 1부터 46까지설정한다.
(초기값으로 할 수 있는 부분을 찾았지만 일단이렇게 실행되니 그대로 진행하는걸로...)
푸시버튼 리스트는 - 배경을 모두 기본값으로 변경하고 setChecked를 False상태로 변경한다.
코드실행화면
지금보니 콤보박스 폰트를 변경하지 않았네...
창 변환
왜 마지막 버튼은 줄어들지 않는가.
사진자료 (title icon)
코드자료
아쉬운 점
cnt 숫자를 세서 메세지박스를 출력하는 부분은 세 함수가 동일한데
그 부분만 함수로 따로 빼서 만들면 훨씬 간편했을 것 같다.
lineEdit처럼 객체.text()로 불러오면 str형식이라는걸 기억하자.
참고사이트
콤보박스 참고링크: https://stackoverflow.com/questions/67656266/adding-int-values-in-combo-box-in-python
콤보박스 텍스트 가져오기 링크: https://www.geeksforgeeks.org/pyqt5-getting-the-text-of-selected-item-in-combobox/
버튼 keep clicked 참고링크: https://stackoverflow.com/questions/11776347/how-can-i-keep-a-qpushbutton-selected-and-then-find-what-is-selected-in-a-grid-o
체크박스 unchecked 참고링크: https://stackoverflow.com/questions/36281103/change-checkbox-state-to-not-checked-when-other-checkbox-is-checked-pyqt
'개발공부 > Pyqt 파이큐티' 카테고리의 다른 글
[PyQt] QtMultimedia 사용해 영상 재생하기 (1) | 2023.05.24 |
---|---|
[PyQt] Scroll Area 공부하기 (0) | 2023.05.09 |
[PyQt] 파이큐티 QTableWidget 공부하기 (0) | 2023.05.07 |
파이큐티공부_1 로그인창 (0) | 2023.05.07 |
[PyQt] 광주 버스안내도 파이큐티로 만들기 (0) | 2023.05.06 |